Peeling Back the Layers: A Comprehensive Look at Bun, the New JavaScript Runtime
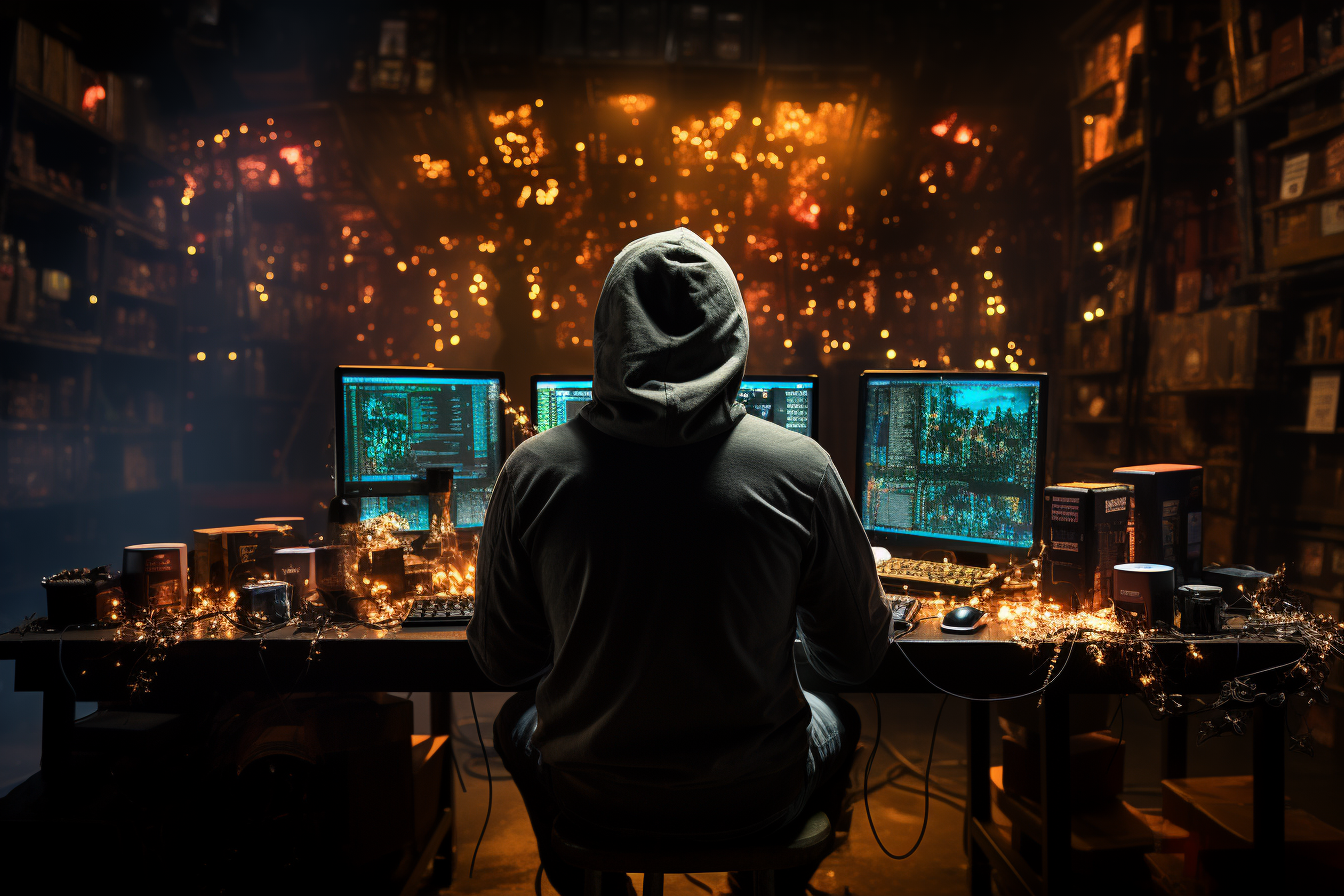
A developer engrossed in coding, much like you could be while exploring the new Bun JavaScript runtime.
Setting the Stage: A Fresh Slice in Web Development
In the ever-evolving world of web development, Bun emerges as the latest JavaScript runtime, promising to rise above the rest with its speed, elegance, and unified developer experience. Developed in Zig—a language that made its debut in 2016—Bun aims to be a direct alternative to Node.js. But does it truly offer a new flavor, or is it just reheating what's already been served? Let's peel back the layers and find out what fills this bun.
The Core: What Exactly is Bun?
Bun is designed to be a drop-in replacement for Node.js, built on the JavaScriptCore engine that powers Safari. While it aims to run a broad spectrum of server-side JavaScript, some critics argue that it's more of an abstraction layer than a groundbreaking innovation. The team behind Bun sets a grand vision for their new product: The goal of Bun is to run most of the world's server-side JavaScript and provide tools to improve performance, reduce complexity, and multiply developer productivity.
The Features: A Mixed Bag
Speed
One of the most compelling features of Bun is its speed. From the moment you boot up a server to the time you execute your tests, Bun is engineered to be fast. This isn't just about shaving milliseconds off your load times; it's about creating a more efficient development cycle. The quicker your tests and deployments run, the faster you can iterate, and the more productive you become.
Optimized APIs
Bun offers a minimal set of APIs, but don't let the word "minimal" fool you. These APIs are highly optimized for common tasks, making it easier to get your project off the ground. Whether you're dealing with file systems, network requests, or data manipulation, Bun aims to provide a streamlined approach that simplifies your code without sacrificing functionality.
Unified Developer Experience
Beyond its core runtime capabilities, Bun aims to offer a cohesive developer experience. It comes with its own package manager, test runner, and bundler. This all-in-one approach eliminates the need to stitch together disparate tools, allowing you to focus on what matters most: building your application.
Windows Support
However, it's not all sunshine and rainbows. Critics have pointed out that Bun's support for Windows is less than stellar, reminiscent of Yarn's early days. While Yarn has since improved its Windows compatibility, the damage was done. Bun risks falling into the same trap if it doesn't address this issue promptly.
Version Management
Another point of contention is Bun's lack of version management. For a tool that aims to be a comprehensive solution for JavaScript development, the absence of this feature is a glaring omission. It's a fundamental aspect that developers rely on for maintaining and scaling their applications.
Ecosystem Lock-in
Lastly, there's the issue of ecosystem lock-in. Features like Macros and bun.x APIs are neat, but they could potentially tie you to Bun's ecosystem, making your code less portable. This could be a significant drawback for teams looking for flexibility and the freedom to switch tools as their project evolves.
The Zig Factor: A Valid Criticism?
Bun's choice of Zig as its development language adds a unique twist. However, it's worth questioning whether this is more about being different than being better. The same performance gains could likely have been achieved with optimized C++. Additionally, the effort spent on Bun could arguably have been used to enhance the existing Node.js ecosystem.
User Perspectives: A Range of Opinions
While some users are excited about Bun's speed and selective feature set, others caution that the tool is still in its infancy. The general consensus? Use Bun, but perhaps not for your most critical projects just yet.
Hands-On: Crafting Your Own Fibonacci API with Bun
Ready to roll up your sleeves and dive into some code? We're going to walk you through creating your own Fibonacci API and a web page to fetch the sequence — all using Bun. While we do have a GitHub repository for reference, we encourage you to follow along and build it yourself for the best learning experience.
Getting Started
Install Bun:
curl -fsSL https://bun.sh/install | bash
Setup a dev env
mkdir fibonacci && cd fibonacci
Step 1: Create the Fibonacci Component
First, let's create a new file named fib.ts. In this file, we'll define a function to generate Fibonacci numbers. We'll use memoization to optimize the function's performance. Here's how you do it:
This function uses an IIFE (Immediately Invoked Function Expression) to create a closure around the memo object, which stores previously computed Fibonacci numbers. This way, we avoid recomputing them, making our function more efficient.
Now that we've got our Fibonacci component set up, let's move on to creating the API and server.
Step 2: Create the API to Serve the Fibonacci Sequence
Next, let's create an API to serve the Fibonacci numbers. Create a new file where you'll define your API. You can name it fib-api.ts. In this file, import the fibonacci function we defined earlier and then set up the API like so:
In this code snippet, we define an object with a fetch method that takes a Request object as its argument. We then parse the URL to check if the request is for the /fibonacci endpoint. If it is, we read the n parameter from the URL's search parameters, calculate the n-th Fibonacci number using our fibonacci function, and return it as a JSON response.
If the function throws an error (which it will if n is negative), we catch that error and return a 400 Bad Request response.
If the URL path doesn't match /fibonacci, we return a 404 Not Found response.
Now that we have our API set up, let's move on to running the server.
Step 3: Create the Server to Serve Our API and Web Page
Now that we have our Fibonacci API, let's create a server to serve both the API and a simple web page. Create a new file and name it fib-server.ts. In this file, import the fibonacciAPI we defined earlier and set up the server like so:
In this code, we define an object with an asynchronous fetch method. This method takes a Request object as its argument and uses the URL to determine what to do:
If the URL path starts with /fibonacci, we delegate the request to our fibonacciAPI. Splitting the API from the server in our demo project amps up modularity and makes the code easier to maintain, even if it means we're doubling up on endpoint definitions.
If the URL path is /, we serve an HTML file (index.html) that we'll create next. We read this file using Bun's native file handling capabilities and return it as a text/html response.
If the URL path doesn't match any of the above, we return a 404 Not Found response.
With this, our server is ready to handle requests for both the Fibonacci API and a web page. Next, let's create the HTML page that will display the Fibonacci sequence.
Step 4: Create the HTML Page to Display the Fibonacci Sequence
To tie everything together, let's create an HTML page that will display the first 1000 numbers of the Fibonacci sequence. Create a new file and name it index.html. Populate it with the following content:
In this HTML file, we define a simple grid layout to display the Fibonacci numbers. We also include a JavaScript script that fetches the Fibonacci numbers from our API and populates the list. The script fetches the numbers asynchronously and measures the time it takes to compute and display all 1000 numbers.
With this, you've successfully created a full-stack application using Bun to display the first 1000 numbers of the Fibonacci sequence. You can now run your Bun server and navigate to http://localhost:3000 to see it in action.
Running Your Bun Server
After you've set up all the components, it's time to run your Bun server and see the Fibonacci sequence in action. To do this, navigate to your project directory in the terminal and execute the following command:
bun fib-server.ts
This command will start the Bun server, and it will begin listening for incoming requests. Once the server is up and running, open your web browser and navigate to http://localhost:3000. You should see the first 1000 numbers of the Fibonacci sequence being displayed on the page, along with the time it took to compute and display them.
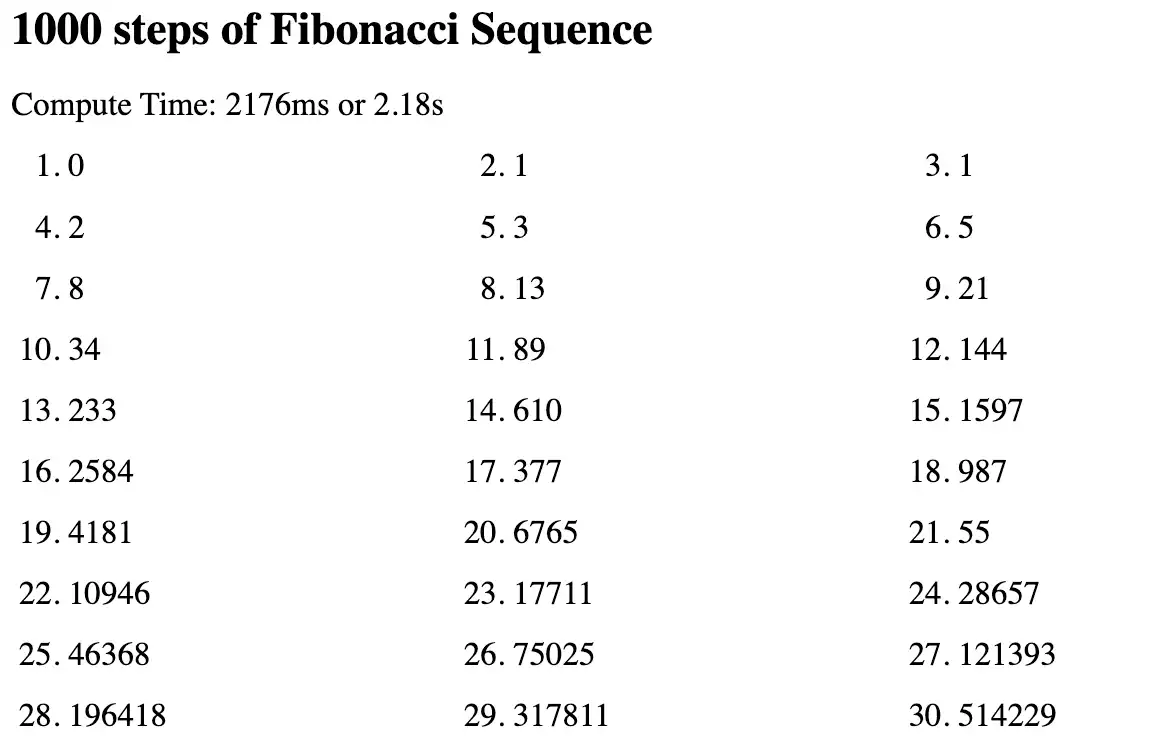
Adding Tests to Your Bun Project
Testing is an essential part of any robust software development process. Bun provides its own testing library, making it easy to write and run tests for your code. Let's add some tests to ensure our Fibonacci function is working as expected.
Create a new file in your project directory and name it fib.test.ts. Inside this file, add the following code:
These tests cover various cases, from basic Fibonacci numbers to edge cases like negative integers. They help ensure that your Fibonacci function behaves as expected under different conditions.
Running Your Tests in Bun
After you've added your tests, it's time to run them and see if your Fibonacci function is working as expected. Open your terminal, navigate to your project directory, and run the following command:
bun test
This will execute all the tests you've written in fib.test.ts. If everything is set up correctly, you should see output indicating whether each test passed or failed. This is a great way to ensure that your code is robust and behaves as expected under different conditions.
The Simplicity of Bun: What We Didn't Do
Before we wrap up, let's take a moment to appreciate the simplicity of working with Bun. Notice what steps were conspicuously absent from this guide:
No npm init to initialize a Node.js package.
No installing a myriad of dependencies or dev dependencies.
No complex build or compile steps.
All you needed was to have Bun installed on your system. From there, you simply wrote your files and ran your server and tests using Bun's built-in commands. It's a streamlined experience that lets you focus more on coding and less on configuration. To me, that is the true value of Bun.
And there you have it — a complete guide to building a Fibonacci API and web page using Bun, complete with tests. This should give you a good sense of what Bun has to offer and how you can use it for your own projects.
Wrapping Up: The Pros, Cons, and Potential of Bun
Bun offers a cohesive developer experience with its speed, elegant APIs, and built-in package manager, test runner, and bundler. However, it's not without its challenges. Critics argue that Bun could have contributed these features to the existing Node.js ecosystem rather than creating a new one. Additionally, the choice to build on Zig, a language that hasn't shown significant advantages over its alternatives, raises questions about Bun's long-term viability.
As Bun has just launched its version 1.0, it's still early days. Whether it becomes a mainstay or not, its presence is likely to influence the broader ecosystem. So, will Bun rise to the occasion as the future of JavaScript runtimes, or will it crumble under the weight of its own ambition? Only time will tell. For now, it's an interesting option that warrants a closer look, but one that should be approached with a clear understanding of its pros and cons.
Happy coding, or should we say, let's get this Bun in the oven!